Husky (Github) is a powerful tool that provides Git hooks for your development workflow. Git hooks are scripts that run automatically at specific moments during the Git workflow, such as before committing code or before pushing changes to a remote repository. Husky simplifies the setup and management of these hooks in your React projects.
Using Husky in React projects brings several benefits. Firstly, it ensures consistent code quality and enforces best practices by running automated checks and tests before committing or pushing code. This helps catch errors, style violations, and other issues early in the development process, leading to cleaner and more reliable code.
Husky also improves collaboration and reduces the risk of merging conflicts by automatically running pre-commit hooks that can check for issues like lint errors or failing tests. This encourages developers to address issues promptly, preventing them from being pushed to the repository and potentially affecting the work of other team members.
Another advantage of Husky is that it provides a simple configuration interface, allowing you to define custom scripts and commands to run as part of the Git workflow. This flexibility enables you to integrate additional tools or perform specific actions tailored to your project’s needs.
In summary, integrating typicode/Husky
into your React projects enhances code quality, promotes collaboration, and streamlines the development process by automating checks and tests at crucial moments of the Git workflow. It is a valuable tool for teams and individual developers who strive for code excellence and smoother project management.
In our projects we use a few different Husky workflows. I’m going to show them below.
Step 1 – Add Husky to your React project
yarn add husky -D
npm pkg set scripts.prepare="husky install"
npx husky install
Check for linting errors
Linting errors in React refer to issues identified by a linting tool that analyze your code for potential errors, style violations, and code quality improvements. These errors are based on predefined rules or configurations established by the linting tool or your project. When working with React, linting errors can include missing or incorrect syntax, unused variables or imports, inconsistent code formatting, and violations of best practices.
I think we want to make sure we don’t have any of such errors eh :).
First, we extend the package.json and add the following line under the scripts section:
"lint": "eslint --ext .ts,.tsx ./src",
If your root folder is different from ‘./src’, please make sure to adjust the script accordingly.
Next, we add a pre-commit script with the following command:
npx husky add .husky/pre-commit "yarn lint"
This makes sure that before every commit we do, the ‘yarn lint’ command is being triggered. It forces you to fix linting errors before you are able to commit code.
Commit message formatting
Tired of people making commits with weird messages that don’t say anything regarding what has been done? No longer! You can force your team to use specific naming conventions.
Add the following packages:
yarn add @commitlint/cli -D
yarn add @commitlint/config-conventional -D
npx husky add .husky/commit-msg "npx --no -- commitlint --edit"
Make sure to add a commitlint.config.js to the root file of your project. We use the following content:
// build: Changes that affect the build system or external dependencies (example scopes: gulp, broccoli, npm)
// ci: Changes to our CI configuration files and scripts (example scopes: Travis, Circle, BrowserStack, SauceLabs)
// docs: Documentation only changes
// feat: A new feature
// fix: A bug fix
// perf: A code change that improves performance
// refactor: A code change that neither fixes a bug nor adds a feature
// style: Changes that do not affect the meaning of the code (white-space, formatting, missing semi-colons, etc)
// test: Adding missing tests or correcting existing tests
module.exports = {
extends: ['@commitlint/config-conventional'],
rules: {
'body-leading-blank': [1, 'always'],
'body-max-line-length': [2, 'always', 100],
'footer-leading-blank': [1, 'always'],
'footer-max-line-length': [2, 'always', 100],
'header-max-length': [2, 'always', 100],
'scope-case': [2, 'always', 'lower-case'],
'subject-case': [2, 'never', ['sentence-case', 'start-case', 'pascal-case', 'upper-case']],
'subject-empty': [2, 'never'],
'subject-full-stop': [2, 'never', '.'],
'type-case': [2, 'always', 'lower-case'],
'type-empty': [2, 'never'],
'type-enum': [
2,
'always',
[
'build',
'chore',
'ci',
'docs',
'feat',
'fix',
'perf',
'refactor',
'revert',
'style',
'test',
'translation',
'security',
'changeset',
],
],
},
};
Try to make a commit now with something like ‘wowoww’. You will see the following error:

When you open the git log, you’ll see the following:
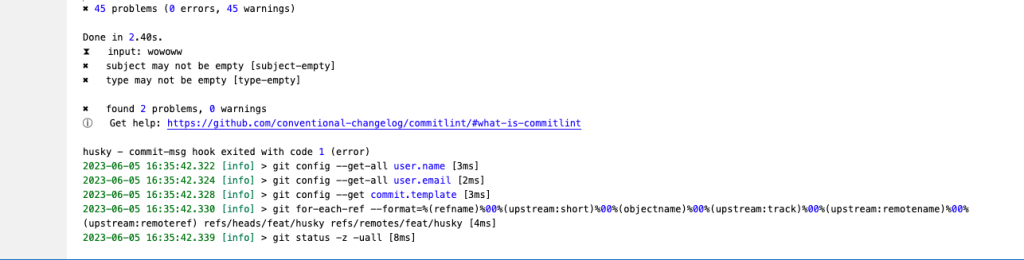
According to the commitlint.config.js you need to prefix your message with one of the following types:
'build',
'chore',
'ci',
'docs',
'feat',
'fix',
'perf',
'refactor',
'revert',
'style',
'test',
'translation',
'security',
'changeset',
So, for example, the following commitmessage would work:
feat: added commit-msg validator
Check if the project builds before being able to push code
We utilize a Husky script to ensure that the build process is validated before pushing code to our Azure DevOps environment.
To add the pre-push script, run the following command:
npx husky add .husky/pre-push "yarn build"
This script executes the ‘yarn build’ command and verifies its success before allowing code to be pushed to the source control. It serves as a safeguard to ensure that only properly built code is pushed, contributing to the stability and reliability of our project.